if-statement and callback:
void Start()
{
if (StatusNavigation.Show(ToggleCompleted))
{
Debug.Log("The system bars are ready and toggling now.");
}
else
{
Debug.Log("The system bars are not ready.");
}
}
void ToggleCompleted()
{
Debug.Log("Toggled!");
}
Apply color transitions to the bars:
// === Status & Navigation ===
StatusNavigation.TransitionColor(Color color, float seconds);
StatusNavigation.TransitionLightMode(float seconds);
StatusNavigation.TransitionDarkMode(float seconds);
// === Status ===
StatusBar.TransitionColor(Color color, float seconds);
StatusBar.TransitionLightMode(float seconds);
StatusBar.TransitionDarkMode(float seconds);
// === Navigation ===
NavigationBar.TransitionColor(Color color, float seconds);
NavigationBar.TransitionLightMode(float seconds);
NavigationBar.TransitionDarkMode(float seconds);
if-statement, color transition and callback:
void ShowStatusBar()
{
// Status Bar Transition Color
Color fromColorTransition = StatusNavigation.target.statusBar.GetComponent<Image>().color;
Color toColorTransition = Color.white;
// Show Status Bar
if (StatusBar.Show(fromColorTransition, toColorTransition, ToggleCompleted))
{
Debug.Log("The status bar is ready and toggling now.");
}
else
{
Debug.Log("The status bar is not ready.");
}
}
void HideStatusBar()
{
// Status Bar Transition Color
Color toColorTransition = Color.white;
// Hide Status Bar
if (StatusBar.Hide(toColorTransition, ToggleCompleted))
{
Debug.Log("The status bar is ready and toggling now.");
}
else
{
Debug.Log("The status bar is not ready.");
}
}
void ToggleCompleted()
{
Debug.Log("Toggled!");
}
Get color of status bar:
Color statusLightColor = StatusNavigation.statusLightModeBackgroundColor;
Color statusDarkColor = StatusNavigation.statusDarkModeBackgroundColor;
Get color of navigation bar:
Color navigationLightColor = StatusNavigation.navigationLightModeBackgroundColor;
Color navigationDarkColor = StatusNavigation.navigationDarkModeBackgroundColor;
Get height of status and navigation bar:
// === Realtime Data ===
float statusBarHeight = StatusNavigation.StatusBarHeight;
float navigationBarHeight = StatusNavigation.NavigationBarHeight;
// === Static Data ===
float staticStatusBarHeight = StatusNavigation.StatusBarHeight_STATIC;
float staticNavigationBarHeight = StatusNavigation.NavigationBarHeight_STATIC;
Other:
UnityEvent backButtonEvent = StatusNavigation.backButtonOnClick;
bool startLightMode = StatusNavigation.startLightMode;
bool statusIsReady = StatusNavigation.IsReadyStatusBar;
bool navigationIsReady = StatusNavigation.IsReadyNavigationBar;
Set system bars during the Splash Screen by editing this C# file:
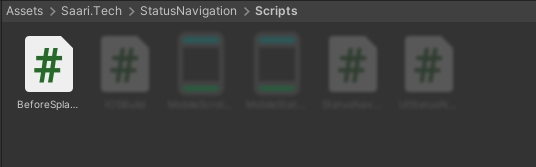
Before using this code, ensure that Hide Navigation Bar option is unchecked in the Resolution and Presentation settings of Android Player Settings and that Status Bar Hidden option is unchecked in the Resolution and Presentation settings of iOS Player Settings.
The provided code, located in the BeforeSplashScreen.cs file, it overrides specific aspects of the system bars during the Splash Screen phase. However, be aware that this code has limited functionality and may not cover all scenarios or requirements.
// === Display ===
internal static bool BeforeSplashScreenDisplayStatusBar = true;
internal static bool BeforeSplashScreenDisplayNavigationBar = true;
// === Light Mode ===
internal static bool BeforeSplashScreenStatusBarLightMode = true;
internal static bool BeforeSplashScreenNavigationBarLightMode = true;
Getting Keyboard Data
To retrieve the current height and width of the mobile keyboard, use the following properties:
float keyboardHeight = MobileKeyboardManager.Height;
float keyboardWidth = MobileKeyboardManager.Width;
Go to Assets/Saari.Tech/StatusNavigation/Scripts/Config.cs and set true to Simple Mode.
internal static bool SimpleMode = true;
Setup:
- Add a MobileKeyboardManager component beside MobileStatusNavigationBar in the Safe Zone.
- Make sure Mobile Status & Navigation bar is running in Simple Mode.
- This method works for both Android and iOS platforms. iOS is currently not tested.
Feel free to use these code snippets to access and manipulate the status and navigation bar properties in your application.